2022/05/25
Laravel ではマイグレーションという便利な機能(データベースを管理する機能)が用意されています。
カラムの追加や削除はマイグレーションファイルで行います。
しかし、毎回、書き方を忘れてしまうんですよね。
そんなわけで、今回はマイグレーションファイルにおけるカラムの設定についてまとめました。
INDEX
書式
基本
例:nameカラムのデータ型を 「varchar」 に設定
$table->string('name');
カラム属性の変更
chengeメソッドは存在するカラムを新しいタイプへ変更するか、カラムの属性を変更します。
例:nameのカラムのサイズを25から50へ変更
$table->string('name', 50)->change();
例:さらにNULL値を設定可能にする
$table->string('name', 50)->nullable()->change();
down() では NULL を許容しない制約を記述することになります。
しかし NULL を禁止するメソッドは用意されていないため、SQL を直接書くことになります。
public function down()
{
Schema::table('users',function(Blueprint $table){
# ---------------
# 5.5 以前
# ---------------
DB::statement('ALTER TABLE users MODIFY name VARCHAR NOT NULL;');
// SQL のバージョンによっては以下のような書き方になることも
DB::statement("ALTER TABLE `users` CHANGE `name` `name` VARCHAR(255) NOT NULL");
# ----------------
# 5.5 以降は以下の記述ができます。
# ----------------
// $table->string('name')->nullable(false)->change();
});
}
}
カラムの削除
ロールバックの項目( down() )でよく使います。
$table->dropColumn('name');
dropColumnメソッド
にカラム名の配列を渡せば、複数のカラムをドロップできます。
$table->dropColumn(['zip', 'country' , 'address']);
カラムの設定
データ型
メソッド | 説明 |
---|---|
increments('id') | 「符号なしINT」を使用した自動増分ID(主キー) |
unsignedBigInteger('user_id') / foreignId('user_id') |
外部キーのカラムを指定する場合は以下のように指定する。
|
boolean('カラム名') |
真偽値カラム ※フラグ関係のカラムはこれを使う
|
integer('カラム名') |
INT型(範囲:-2147483647〜2147483647 4バイト)
|
tinyInteger('カラム名') | TINYINT型(範囲:0~255 1バイト) |
bigInteger('カラム名') | BIGINT型 |
string('カラム名') | VARCHAR型 ※デフォルト255文字
|
string('カラム名', 長さ) |
長さ指定の VARCHAR カラム
|
timestamps() | created_at と update_at カラム
|
text('カラム名') | TEXT型
|
softDeletes() |
ソフトデリートのためのNULL値可能な deleted_at カラム追加
|
int型の入力可能桁数
型 | バイト | 最小値 | 最大値 |
---|---|---|---|
TINYINT | 1 | -128 | 127 |
SMALLINT | 2 | -32768 | 32767 |
MEDIUMINT | 3 | -8388608 | 8388607 |
INT | 4 | -2147483648 | 2147483647 |
BIGINT | 8 | -9223372036854775808 | 9223372036854775807 |
int(11)の 11 は、カラムの表示幅であり、データベースの運用上はあまり意味がありません。intのバイト数は、11 を 10 や 8 に変更しても4バイトであり、結局、2147483647まで格納が可能。
テキストデータ
type | 最大サイズ |
---|---|
varchar | VARCHAR の有効な最大長は、最大行サイズは65,535バイトです。UTF8を使用している場合は、1文字が最大3バイトになり、VARCHARに登録できる文字数は最大21,844文字となります。 |
text | 最大長が 65,535文字のテキストカラム。 |
MEDIUMTEXT | 文字型ラージオブジェクトカラム、最大長は 16,777,215 文字。 |
LONGTEXT | 文字型ラージオブジェクトカラム、最大長は 4,294,967,295 文字。 |
※ MySQL 4.1より前は、varchar(n)はバイト数でしたが、MySQL 4.1以降は、ユニコード等の実装に伴いマルチバイトも1文字とカウントする文字数になっています。つまり、varchar(255) は255文字。
オプション(カラム修飾子)
オプション | 説明 |
---|---|
comment('コメント内容') |
カラムにコメントを追加
|
nullable() |
カラムにNULL値を許す
|
unsigned() |
符号なし(unsigned)属性を付与する。
|
default('値') |
カラムのデフォルト値を設定
|
after('カラム名') |
指定したカラムの次にカラムを追加します。 (※ MySQLのみの仕様です。)
|
インデックス
インデックス | 説明 |
---|---|
index('カラム名') |
カラムにインデックスを追加
|
unique('email') |
カラムにユニークキーを追加
|
unsigned() |
符号なし(unsigned)属性を付与する。
|
外部キーの作成
例:rolesテーブルの user_id を外部キーとしてusersテーブルと紐づける。
外部キーを設定しておくと、rolesテーブルの user_id
にusersテーブルの id
が自動で入ってくれます。
public function up()
{
Schema::table('roles', function (Blueprint $table){
$table->integer('user_id')->unsigned()->change(); // 符号無し属性に変更
$table->foreign('user_id')->references('id')->on('users'); // 外部キー参照
});
}
increments()で作ったusersテーブルのidカラム
にはunsined(符号無し)属性が付与されます。
役割テーブル(roles)のuser_id
にも同様の制約をつけないと形式の一致でエラーになります。
実例
public function up()
{
Schema::create('users', function(Blueprint $table)
{
$table->increments('id', true)->unsigned()->comment('ID');
$table->string('family_name', 40)->comment('姓');
$table->string('first_name', 40)->comment('名');
$table->string('email')->unique('email')->comment('メールアドレス');
$table->string('password')->comment('パスワード');
$table->date('birthdate')->comment('生年月日');
$table->tinyInteger('gender')->unsigned()->comment('性別 : 1 : 男性 2 : 女性');
$table->string('zip', 10)->comment('郵便番号');
$table->tinyInteger('prefecture_code')->unsigned()->comment('都道府県コード : JIS都道府県コード');
$table->string('prefecture', 40)->comment('都道府県名 フリーテキスト検索で使用');
$table->string('address')->comment('住所2');
$table->string('tel', 20)->nullable()->comment('電話番号');
$table->text('memo', 65535)->nullable()->comment('メッセージ');
$table->rememberToken();
$table->timestamps();
$table->softDeletes()->comment('削除日時 : 削除・退会を行った日時 この値がnullでなかったら、削除・退会を行ったとみなす');
});
}
また、何か発見したら追記していこうと思います。
以上です。
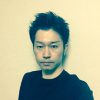
仕事で Laravel を使っています。気づいたことや新しい発見など情報を発信していきます。問い合わせはこちら。