2019/08/26
Laravelを使ってDBにある情報をCSVでダウンロードする機能を作成するシリーズです。
前回はイントロをエントリーしました。
今回はテーブルを作成してサンプルデータを入れるところまでやります。具体的には
・マイグレーション
・モデル
・シーダー
・モデル
・シーダー
の作成をやります。
手順
1.マイグレーションファイルの作成&実行
マイグレーションファイルのスケルトン作成
// 認証用テーブルの作成
php artisan make:migration create_auth_information_table --create=auth_information
// プロフィール用テーブルの作成
php artisan make:migration create_profiles_table --create=profiles
マイグレーションファイルの編集
***_create_auth_information_table.php
public function up()
{
Schema::create('auth_information', function (Blueprint $table) {
$table->increments('id');
$table->integer('authinformation_id')->after('id');
$table->string('email')->unique();
$table->string('password');
$table->rememberToken();
$table->timestamps();
});
}
***_create_profiles_table.php
public function up()
{
Schema::create('profiles', function (Blueprint $table) {
$table->increments('id');
$table->string('name')->comment('なまえ');
$table->string('address')->comment('住所');
$table->date('birthdate')->comment('生年月日');
$table->string('tel')->comment('電話番号');
$table->text('msg')->comment('メッセージ');
$table->timestamps();
});
}
マイグレーションファイルの実行
php artisan migrate
マイグレーションファイルに関しての基本的な解説は以下をご覧ください。
2.モデルの作成
モデルのスケルトンを作成
// 認証用
php artisan make:model Models\AuthInformation
// プロフィール用
php artisan make:model Models\Profile
モデルの編集
認証用(AuthInformation.php)
class AuthInformation extends Model
{
//テーブル名を変更したい
protected $table = 'auth_information';
//ブラックリスト方式
protected $guarded = ['id'];
}
プロフィール用
class Profile extends Model
{
//ブラックリスト方式
protected $guarded = ['id'];
}
モデルの作成に関しての基本的な解説は以下をご覧ください。
3.シーダーファイルの作成&実行
シーダーファイルのスケルトン作成
// 認証用
php artisan make:seed AuthInformationTableSeeder
//プロフィール用
php artisan make:seed ProfileTableSeeder
シーダーファイルの編集
認証用(AuthInformationTableSeeder.php)
public function run()
{
// 一旦テーブル削除
DB::table('auth_information')->delete();
// faker使う
$faker = Faker\Factory::create('ja_JP');
// レコード100人分出力
for($i=0; $i < 100; $i++){
\App\Models\AuthInformation::create([
'email' => $faker->email(),
'password' => $faker->password(),
]);
}
}
プロフィール用(ProfileTableSeeder.php)
public function run()
{
//一旦削除
DB::table('profiles')->delete();
// faker使う
$faker = Faker\Factory::create('ja_JP');
// レコード100人分出力
for($i=0; $i < 100; $i++){
\App\Models\Profile::create([
'authinformation_id' => $i+601,
'name' => $faker->name(),
'address' => $faker->address(),
'birthdate' => $faker->dateTimeBetween('-80 years', '-20years')->format('Y-m-d'),
'tel' => $faker->phoneNumber(),
'msg' => $faker->text()
]);
}
}
シーダーファイルの実行
php artisan db:seed
シーターについての基本的な解説は以下をご参照ください。
ステップ1は以上です。
次回はステップ2(ビューの作成)をやります。
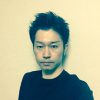
仕事で Laravel を使っています。気づいたことや新しい発見など情報を発信していきます。問い合わせはこちら。