2018/10/12
共通レイアウトを利用して各ページを作成していきます。
目標
各ページのビューファイルを作成
予備知識
Bladeの使い方は理解しておく必要があります。
入力データを残すold()やフォームアクションの指定でurl()を使います。
フォームの作成にはCSRF対策が必要なので事前にこの辺りも勉強しておきましょう。
更新や削除のフォームには隠しフィールド(hidden)に_methodプロパティを指定します。
進め方
【1】一覧画面の作成
【2】詳細画面の作成
【3】新規作成画面の作成
【4】編集画面の作成
【1】一覧画面の作成
一覧で表示させる項目は
- 名前
- メールアドレス
だけにします。
@extends('layouts.master_crud') @section('title', '一覧表示') @section('content') @if (session('status')) <div class="alert alert-success" role="alert" onclick="this.classList.add('hidden')"> {{ session('status') }} @if (session('status'))<p>{{session('message')}}</p>@endif </div> @endif <!--↓↓ 検索フォーム ↓↓--> <form method="get" class="form-inline text-right" action="{{url('/')}}/crud" style="margin: 15px 0;"> <div class="form-group"> <input type="text" name="keyword" value="{{$keyword}}" class="form-control" placeholder="名前を入力してください"> </div> <input type="submit" value="検索" class="btn btn-info"> </form> <div class="paginate text-right"> {{ $data->appends(Request::only('keyword'))->links() }} </div> <table class="table table-striped"> <!-- loop --> @foreach($data as $val) <tr> <td><a href="{{ action('CrudController@show', $val->id) }}">{{$val->name}}</a></td> <td>{{$val->mail}}</td> <td><a href="{{ action('CrudController@edit', $val->id) }}" class="btn btn-primary btn-sm">編集</a></td> <td> <form action="{{ action('CrudController@destroy', $val->id) }}" id="form_{{ $val->id }}" method="post"> {{ csrf_field() }} {{ method_field('delete') }} <a href="#" data-id="{{ $val->id }}" class="btn btn-danger btn-sm" onclick="deletePost(this);">削除</a> </form> </td> </tr> @endforeach </table> <div class="paginate text-right"> {{ $data->appends(Request::only('keyword'))->links() }} </div> <!-- /************************************ 削除ボタンを押してすぐにレコードが削除 されるのも問題なので、一旦javascriptで 確認メッセージを流します。 *************************************/ //--> <script> function deletePost(e) { 'use strict'; if (confirm('本当に削除していいですか?')) { document.getElementById('form_' + e.dataset.id).submit(); } } </script> @endsection
【2】詳細画面の作成
@extends('layouts.master_crud') @section('title', '詳細ページ') @section('content') <table class="table table-striped"> <tr> <th>お名前</th> <td>{{$user->name}}</td> </tr> <tr> <th>メールアドレス</th> <td>{{$user->mail}}</td> </tr> <tr> <th>性別</th> <td>{{$user->gender}}</td> </tr> <tr> <th>年齢</th> <td>{{$user->age}}</td> </tr> <tr> <th>都道府県</th> <td>{{$user->pref}}</td> </tr> <tr> <th>誕生日</th> <td>{{$user->birthday}}</td> </tr> <tr> <th>電話番号</th> <td>{{$user->tel}}</td> </tr> </table> @endsection
【3】新規作成画面の作成
入力した文字がバリデーション時に消えないようにoldヘルパーを埋め込んでおきます。
Bladeテンプレートでは中括弧2つで echo を意味します。(※ちなみに中括弧3つでエスケープ処理をします。)
<input type="text" name="name" id="name" value="{{ old('name') }}" class="form-control" placeholder="名前を文字を入力してください" autofocus>
チェックボックスやセレクトボックスの場合はoldヘルパーの値を条件分岐させます。
条件式にoldヘルパーを使うので先ほどのように中括弧を囲むとエラーになるので注意してください。
<input type="radio" name="gender" value="男性" @if(old('gender')!='女性')checked="checked"@endif>男性 <input type="radio" name="gender" value="女性" @if(old('gender')=='女性')checked="checked"@endif>女性
新規作成画面のソースは以下になります。
@extends('layouts.master_crud') @section('title', '新規作成') @section('content') @if (session('status')) <div class="alert alert-success" role="alert" onclick="this.classList.add('hidden')">{{ session('status') }}</div> @endif <p style="margin: 15px 0;">{{$message}}</p> <form class="form-horizontal" role="form" method="post" action="{{url('/crud')}}"> <input type="hidden" name="_token" value="{{csrf_token()}}"> <div class="form-group"> <label for="name" class="control-label col-sm-2">名前:</label> <div class="col-sm-10"> <input type="text" name="name" id="name" value="{{ old('name') }}" class="form-control" placeholder="名前を文字を入力してください" autofocus> @if($errors->has('name')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('name') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"> <label for="email" class="control-label col-sm-2">メールアドレス:</label> <div class="col-sm-10"> <input type="email" name="mail" id="mail" class="form-control" placeholder="メールアドレスを入力してください" value="{{ old('mail') }}" autofocus> @if($errors->has('mail')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('mail') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"><!--※.horizontalではラジオボタンをform-groupで囲む--> <label class="control-label col-sm-2">性別</label> <div class="col-sm-10"> <label class="radio-inline"> <input type="radio" name="gender" value="男性" @if(old('gender')!='女性')checked="checked"@endif>男性 </label> <label class="radio-inline"> <input type="radio" name="gender" value="女性" @if(old('gender')=='女性')checked="checked"@endif>女性 </label> </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"> <label for="age" class="control-label col-sm-2">年齢:</label> <div class="col-sm-10"> <input type="number" name="age" id="age" value="{{ old('age') }}" class="form-control" placeholder="年齢を入力してください" autofocus> @if($errors->has('age')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('age') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"> <label for="pref" class="control-label col-sm-2">都道府県:</label> <div class="col-sm-10"> <select name="pref" id="pref" class="form-control"> <option value="">都道府県の選択</option> <option value="北海道" @if( old('pref')=='北海道') selected @endif>北海道</option> : ※省略 <option value="沖縄県" @if( old('pref') =='沖縄県') selected @endif>沖縄県</option> </select> @if($errors->has('pref')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('pref') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"> <label for="birth" class="control-label col-sm-2">生年月日:</label> <div class="col-sm-10"> <input type="date" name="birthday" id="birthday" class="form-control" placeholder="生年月日を入力してください" value="{{ old('birthday') }}" autofocus> @if($errors->has('birthday')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('birthday') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <div class="form-group"> <label for="tel" class="control-label col-sm-2">電話:</label> <div class="col-sm-10"> <input type="text" name="tel" id="tel" class="form-control" placeholder="電話番号を入力してください" value="{{ old('tel') }}" autofocus> <p id="phone-help" class="help-block">ハイフン"-"なしで数字だけ入力してください (例 09077557720)</p> @if($errors->has('tel')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('tel') }}</p> @endif </div><!--/.col-sm-10--> </div><!--/.form-group--> <button class="btn btn-lg btn-primary btn-block" type="submit">送信</button> </form> @endsection
【4】編集画面の作成
編集画面でもoldヘルパーを使います。
第一引数にはname属性を指定し、第二引数にはDBのデータを指定します。
<input type="text" name="name" value="{{ old('name', $data->name) }}" id="name" class="form-control" placeholder="名前を文字を入力してください" autofocus>
DBにデータがあればそれを表示し、なければ直前の入力データを表示させます。
@extends('layouts.master_crud') @section('title', '編集ページ') @section('content') <p>{{$message}}</p> <form class="form-horizontal" role="form" method="post" action="{{url('/crud',$data->id)}}"> <input type="hidden" name="_token" value="{{csrf_token()}}"> {{-- 隠しフィールド --}} <input type="hidden" name="_method" value="PATCH"> <!--↓↓名前↓↓--> <div class="form-group"> <label for="name" class="control-label col-sm-2">名前:</label> <div class="col-sm-10"> <input type="text" name="name" value="{{ old('name', $data->name) }}" id="name" class="form-control" placeholder="名前を文字を入力してください" autofocus> @if($errors->has('name')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('name') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑名前↑↑--> <!--↓↓メールアドレス↓↓--> <div class="form-group"> <label for="email" class="control-label col-sm-2">メールアドレス:</label> <div class="col-sm-10"> <input type="email" name="mail" value="{{ old('mail', $data->mail) }}" id="email" class="form-control" placeholder="メールアドレスを入力してください" autofocus> @if($errors->has('mail')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('mail') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑メールアドレス↑↑--> <!--↓↓性別↓↓--> <div class="form-group"><!--※.horizontalではラジオボタンをform-groupで囲む--> <label class="control-label col-sm-2">性別</label> <div class="col-sm-10"> <label class="radio-inline"> <input type="radio" name="gender" value="男性" @if(old('gender',"$data->gender")!='女性')checked="checked"@endif>男性 </label> <label class="radio-inline"> <input type="radio" name="gender" value="女性" @if(old('gender',"$data->gender")=='女性')checked="checked"@endif>女性 </label> <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑性別↑↑--> <!--↓↓年齢↓↓--> <div class="form-group"> <label for="age" class="control-label col-sm-2">年齢:</label> <div class="col-sm-10"> <input type="number" name="age" id="age" value="{{ old('age', $data->age) }}" class="form-control" placeholder="年齢を入力してください" autofocus> @if($errors->has('age')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('age') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑年齢↑↑--> <!--↓↓都道府県↓↓--> <div class="form-group"> <label for="pref" class="control-label col-sm-2">都道府県:</label> <div class="col-sm-10"> <select name="pref" id="pref" class="form-control"> <option value="">都道府県の選択</option> <option value="北海道" @if( old('pref',"$data->pref")=='北海道') selected @endif>北海道</option> : ※省略 <option value="沖縄県" @if( old('pref',"$data->pref") =='沖縄県') selected @endif>沖縄県</option> </select> @if($errors->has('pref')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('pref') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑都道府県↑↑--> <!--↓↓生年月日↓↓--> <div class="form-group"> <label for="birth" class="control-label col-sm-2">生年月日:</label> <div class="col-sm-10"> <input type="date" name="birthday" id="birthday" value="{{ old('birthday',$data->birthday) }}" class="form-control" placeholder="生年月日を入力してください" autofocus> <p id="phone-help" class="help-block">記入例: 1937/12/26</p> @if($errors->has('birthday')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('birthday') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑生年月日↑↑--> <!--↓↓電話↓↓--> <div class="form-group"> <label for="tel" class="control-label col-sm-2">電話:</label> <div class="col-sm-10"> <input type="number" name="tel" id="tel" value="{{ old('tel',$data->tel) }}" class="form-control" placeholder="電話番号を入力してください" autofocus> <p id="phone-help" class="help-block">記入例: 09077557720</p> @if($errors->has('tel')) <p class="text-danger" style="margin-bottom: 30px;">{{ $errors->first('tel') }}</p> @endif <!--/.col-sm-10--></div> <!--/.form-group--></div> <!--↑↑電話↑↑--> <button class="btn btn-lg btn-primary btn-block" type="submit">送信</button> </form> @endsection
ビューファイルの作成は以上です。
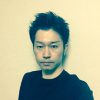
仕事で Laravel を使っています。気づいたことや新しい発見など情報を発信していきます。問い合わせはこちら。